Welcome to the next episode in our GitHub for Beginners series, where we’re diving into the world of GitHub Copilot. This is our sixth episode, and we’ve covered quite a lot of ground. You can check out all our previous episodes on our blog or as videos.
Today we’re going to use GitHub Copilot to help us build a frontend project using React. In the previous episode, we created a backend API for the travel itinerary builder, Planventure. We’ll continue that work and create a React client that leverages our API to interact with Planventure. To see a full description of what we’ll be building, go to this repository and switch to the client-start branch to get started.
What you’ll need
Before we get started, here’s what you’ll need:
- A code editor like VS Code
- The latest version of Node.js
- A package manager like npm
- Access to GitHub Copilot — sign up for free!
Alternatively, you can use a GitHub Codespace to build in the cloud—you’ll still need Copilot access if you’re using a codespace. You can click the Open in GitHub Codespaces button in the repo.
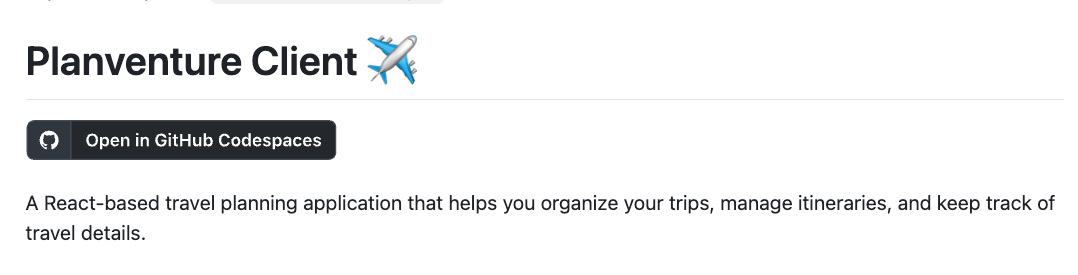
What we’re building
We’re creating a frontend app that connects to the backend API we created in the previous episode. We’ll be using the React library, so it’s recommended that you’re familiar with using React to build client side applications. More specifically, we’ll be using:
- React with Vite for the client.
- Material UI as the component library.
Our goal is to build a working frontend app that has the following features:
- Authenticate users
- Add protected routes
- Add trips and itinerary information
- Edit existing itineraries
Let’s get started!
Step 1: Initial setup
Before we get started, we need to create the appropriate working environment.
- Clone the Planventure repository by opening your terminal in your code editor and running the following command.
git clone https://github.com/github-samples/planventure
- Navigate to the planventure-client directory and switch to the
client-start
branch.
cd planventure-client
git switch client-start
- Install necessary dependencies.
npm install
- Start the server.
npm run dev
- Open a browser to http://localhost:5173 to verify the app is running.
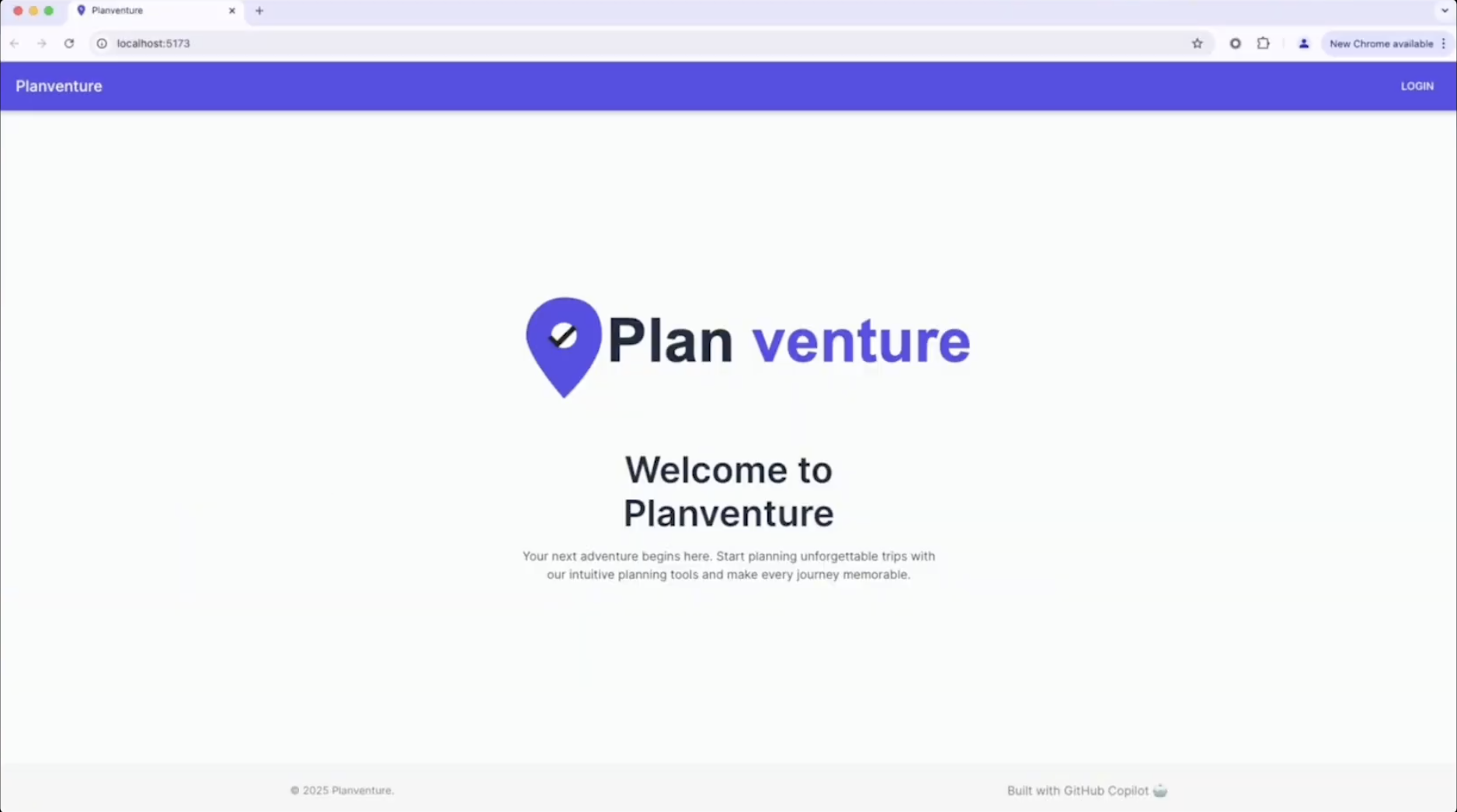
- Become familiar with the code by examining the existing files. Note that some basic components have already been installed and configured. Open Copilot Chat and send it the following prompt to get a basic summary of the existing code:
@workspace Tell me about the configuration setup in the react app.
Now that the initial setup is complete, take a look at the GitHub issue to read detailed information about what we need to build.
Step 2: Create login and registration forms
The first thing we need to add is authentication. We’ll do this by building login and registration forms. But first, we need to create an AuthLayout component to use for all authenticated routes.
- Open Copilot Chat and use the model selector to select the Claude Sonnet 3.5 model.
- Send Copilot the following prompt.
@workspace Create AuthLayout component with navigation and centered content.
- Hover over the proposed solution, click …, and then select Insert into New File.
- Review the added code and save the file. You should always review the code provided by Copilot so that you understand what it is doing and make sure it addresses your prompt.
Now that we’ve created the AuthLayout component, it’s time to build a login form to implement it.
- Send the following prompt to Copilot Chat.
@workspace Build a LoginForm component with email/password fields and validation.
- Create a new folder named auth under the src/components folder.
- Navigate back to Copilot Chat, hover over the proposed solution, click …, and then select Insert into New File.
- Review the added code and save the file in the auth folder.
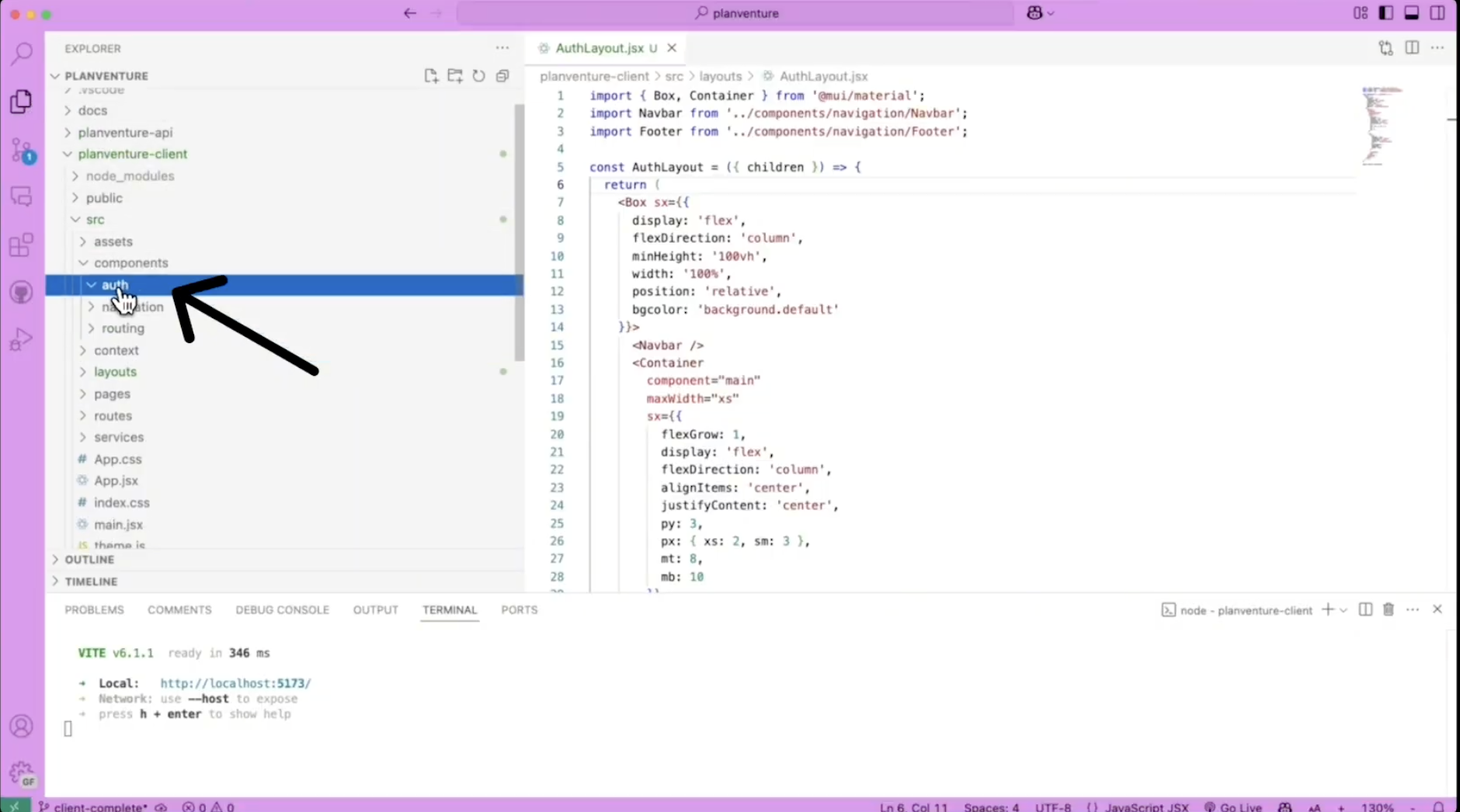
- Return to Copilot Chat and choose Edits from the dropdown.
- Use the Add Files button to add the following files if they are not already listed in the working set:
- AuthLayout.jsx
- LoginForm.jsx
- Routes.jsx
- Send Copilot Edits the following prompt.
Create a new loginPage. Update route and authLayout as needed.
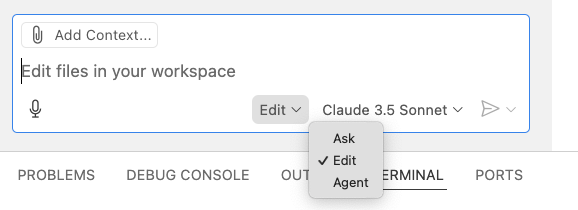
- Review and accept all the changes from Copilot Edits. Don’t forget to save your files.
- Send the following prompt to Copilot Edits to update the Home component.
Update the navbar to use the new loginpage and add a get started button to the home page that routes to the login page.
- Review the code and make any necessary changes. Then save all of the updated files.
- Navigate back to the browser page and refresh it to see the latest changes.
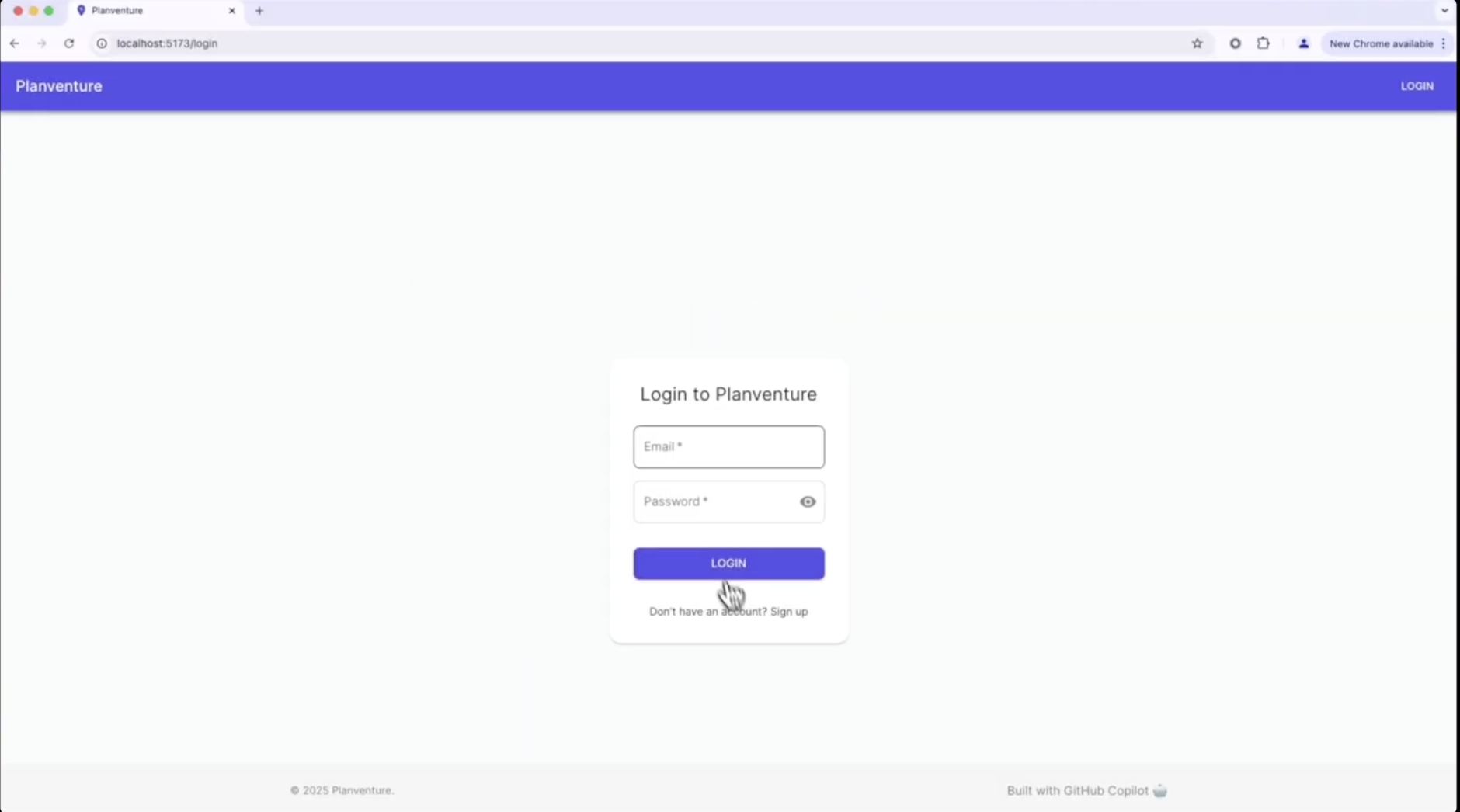
- Commit your changes to the repository. You can use Copilot to automatically generate a commit message by clicking the sparkle button in the top-right corner of the commit message box.
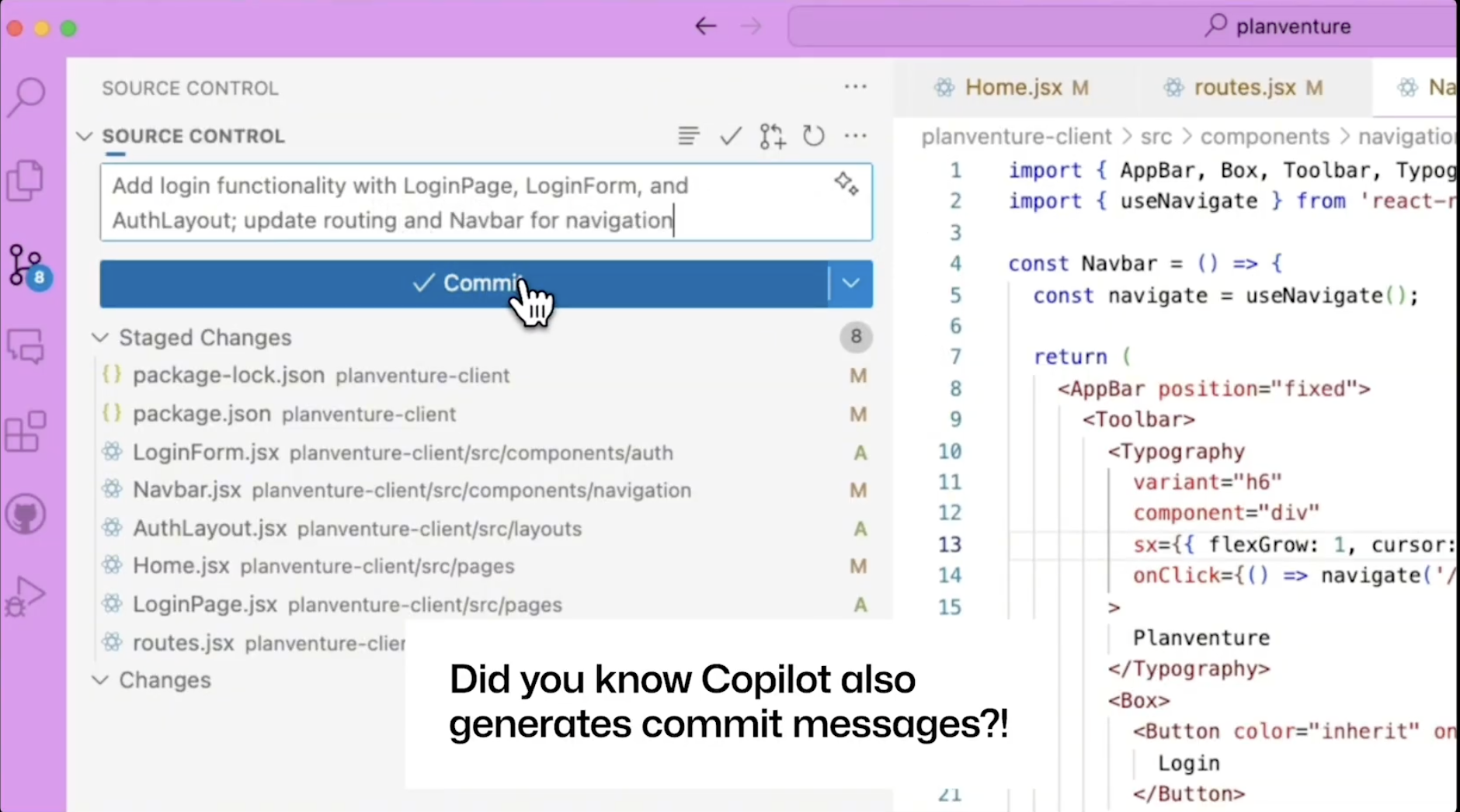
Now we have a Get started button on the UI and the login page. Next we need to create a sign up page so that new users can register.
- Open up Copilot Chat and send it the following prompt.
@workspace Create SignupForm component matching the login form style and a new SignUpPage. Be sure to update routing.
- Hover over the proposed solution to add a SignupForm component in the auth folder, click …, and then select Insert into New File.
- Hover over the proposed solution to add a SignupPage component in the pages folder, click …, and then select Insert into New File.
- For each file that has proposed changes:
- Open the relevant file in your editor.
- Hover over the proposed changes to the file, and click the Apply in editor button.
- Review and accept the changes.
- Save the file.
- Refresh your browser and test the new pages.
- Commit your changes.
For the final piece to this part of the puzzle, we need to update the AuthContext.jsx file.
- Open the AuthContext.jsx file in your editor. It’s located in the context folder.
- Open Copilot Chat and send it the following prompt.
Setup authentication context and token management #file:ProtectedRoute.jsx
- For each file that has proposed changes:
- Open the relevant file in your editor.
- Hover over the proposed changes to the file, and click the Apply in editor button.
- Review and accept the changes.
- Save the file.
- Commit your changes.
Step 3: Use the API for authentication
Now that we have the necessary forms, it’s time to make requests to the backend server so users can register and log in. To do this, we’re going to need to edit three files at once. Luckily, Copilot Chat supports editing multiple files at the same time!
- Send Copilot Chat the following prompt.
@workspace Setup api service functions for login and register routes. Update login and signup forms to use api service. #file:SignupForm.jsx
- For each file that has proposed changes:
- Open the relevant file in your editor.
- Hover over the proposed changes to the file, and click the Apply in editor button.
- Review and accept the changes.
- Save the file.
- Create a new terminal window and cd into the planventure-api directory, then start the server by running the following command:
flask run --debug
- Refresh your browser and try logging into the app . If you run into an error, attempt to debug it with GitHub Copilot. Review the video version of this episode to see some examples of possible things you might need to debug.
- Try signing up a new user and debug any errors that come up.
Now that we’ve verified that we can log in and create new users, we want to add the ability to log out into the navigation bar.
- Send Copilot Chat the following prompt.
@workspace update navbar to include the logout function
- For each file that has proposed changes:
- Open the relevant file in your editor.
- Hover over the proposed changes to the file, and click the Apply in editor button.
- Review and accept the changes.
- Save the file.
- Head back to the browser, refresh it, and test the Logout button. Note that you need to be logged in to see the Logout button.
- Commit all of these changes to your repository.
Congratulations! You’ve now successfully added user authentication!
Step 4: Add a dashboard and sidebar navigation
To ensure that users have easy access to common functionality, we want to create a dashboard with sidebar navigation.
- Click the Copilot button at the top of the window and select Open Copilot Edits.
- Use the Add Files button to add the following files if they are not already listed in the working set:
- App.jsx
- AuthContent.jsx
- AuthLayout.jsx
- LoginForm.jsx
- Navbar.jsx
- SignupForm.jsx
- Send Copilot Edits the following prompt.
Build a dashboard layout component with sidebar navigation.
- Review and accept the suggested code changes, then save the files.
- Refresh your browser to see the new dashboard.
Now that we have a layout, we need to create a TripCard and TripList component to showcase the user’s trips.
- Send the following prompt to Copilot Edits to create the necessary components.
Create TripCard and TripList components for displaying trips with loading states.
- Review and accept the suggested code changes.
- Send the following prompt to add a dashboard component displaying the user’s trips.
Create a dashboard component that displays the trips that users are routed to on login.
- Review and accept the suggested code changes.
- Users should receive a welcome message if they don’t have any trips. They also should get a message if there are any errors when they login. To add these elements, send the following prompt:
Update the dashboard coponent to show a welcome message to users if they have no trips. If there is an error receiving trips, display the image with a message that's quirky and apologetic.
- Review and accept the suggested code changes.
- Now let’s fetch our user trips from the API. Send the following prompt to Copilot Edits:
Update to fetch trips from the flask api.
- Review and accept the suggested code changes, then save the files.
- Return to your browser and refresh the page.
- Test the new functionality by logging in. You should see your trips, a welcome message, or a fun and quirky error message. Try to test all three possibilities.
- Once you’ve verified these changes are working (after debugging any errors that crop up), commit your changes to the repository.
Once we have this piece working, we’re almost there!
Step 5: Enable trip management
Now we need to add the ability for users to manage their trips. In order to do this, we’ll need to start by creating a new form that allows users to add their trips and save them to the dashboard. Then we’ll need to make a POST request to the /trips/new
route.
- Open up your terminal and install the necessary dependencies.
npm install dayjs @mui/x-date-pickers
- Open up Copilot Edits and send it the following prompt.
Create NewTripForm with destination and date inputs. Use the dayjs library.
- Review and accept the suggested code changes, then save the files.
- Navigate back to your browser and refresh the page.
- Click the ADD NEW TRIP button and test the form.
- Commit your changes.
Next up, we want to add the ability for users to manage their itineraries.
- Send Copilot Edits the following prompt.
Create ItineraryDay and TimeSlot components for managing daily activities with editing capabilities.
- Review and accept the suggested code changes, then save the files.
- Send the following prompt to Copilot to encourage users to provide itineraries for their trips.
If the user doesn't have an itinerary for their trip, prompt them to add an itinerary. Also include a default itinerary template.
- Review and accept the suggested code changes, then save the files.
- Return to your browser and refresh the page.
- Click VIEW DETAILS for a trip, then click the ITINERARY tab. You should see a prompt encouraging you to create an itinerary for this trip. Try adding an itinerary, and verify that the app provides a default template.
- Navigate back to Copilot Edits and send the following prompt to give users the ability to edit their trips.
Allow users to edit their trip details.
- Review and accept the suggested code changes, then save the files.
- Return to your browser and refresh the page.
- Try editing a trip and verify that you can change the details.
- Commit your changes.
As a finishing touch, let’s add the ability for users to add additional information about where they’ll be staying and how they’ll be traveling.
- Send the following prompt to Copilot Edits.
Allow users to add their accommodations and transportation details to the Overview page.
- Review and accept the suggested code changes, then save the files.
- Return to your browser, refresh the page, and test this new functionality.
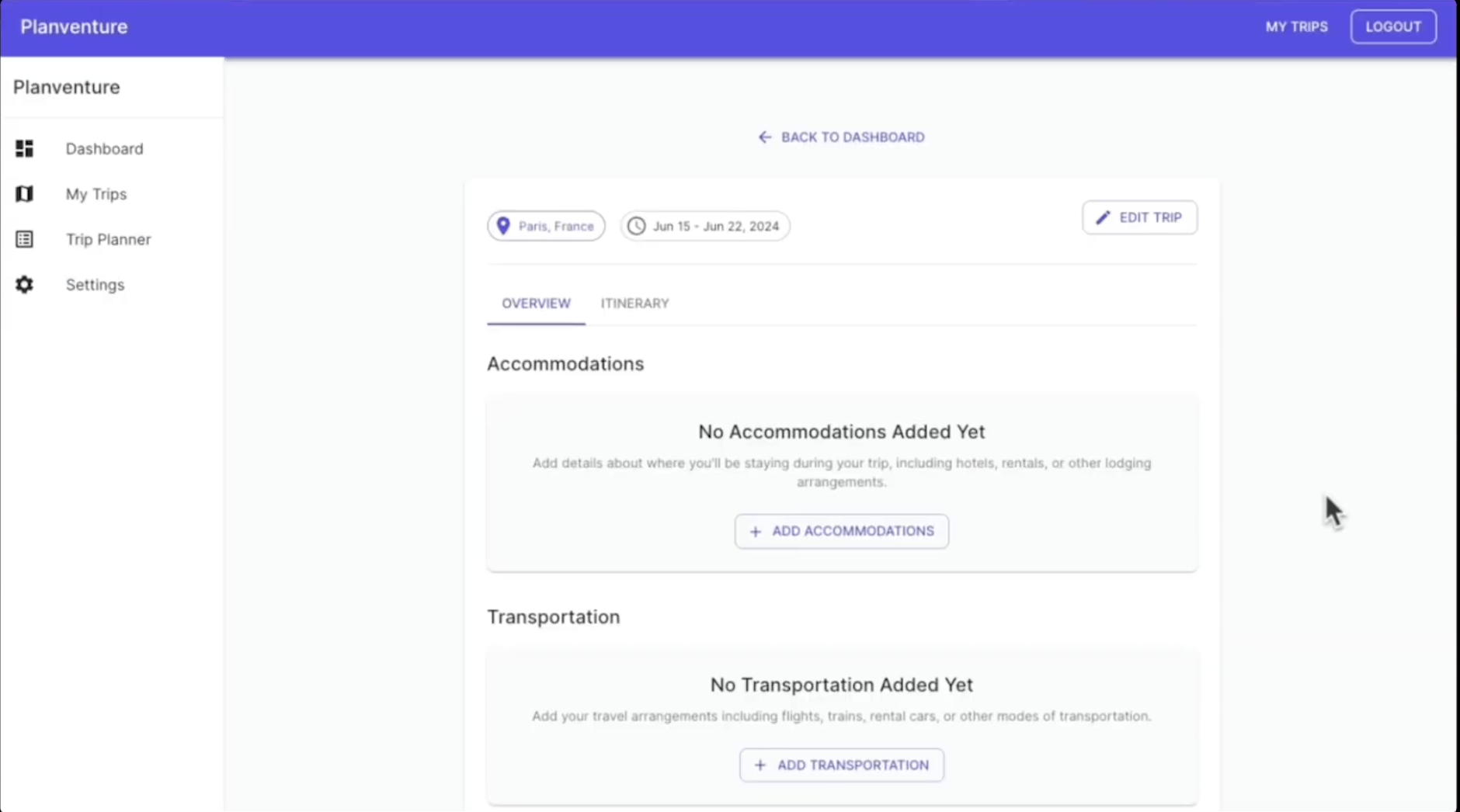
- Don’t forget to commit your changes!
Your next steps
Impressive! You’ve now built a fully functional MVP of the application in a short time, thanks to GitHub Copilot. This is the power of an AI tool that just works.
Don’t forget that you can use GitHub Copilot for free! If you have any questions, pop them in the GitHub Community thread, and we’ll be sure to respond. Join us for the next part in this series, where we’ll build a full app using this API we created.
Happy coding!
The post GitHub for Beginners: Building a React App with GitHub Copilot appeared first on The GitHub Blog.
Source: Read MoreÂ