Understanding and managing errors in automation scripts is crucial for testers. Selenium and Appium are popular tools used for automating tests on web and mobile applications. Familiarity with common Selenium WebDriver exceptions can greatly assist in diagnosing and resolving test failures. Imagine you made a smooth Selenium script. When you run it, you see a WebDriverException error message that is hard to understand. This means there’s a problem with how your test script connects to the web browser and it stops your automated test from working. But don’t worry! If we learn about WebDriverException and why it happens, we can handle these errors better. In this blog, we will talk about what WebDriverException means and share helpful tips to handle it well.
Defining WebDriverException in Selenium
WebDriverException is a common error in Selenium WebDriver. As mentioned earlier, it happens when there is a problem with how your script talks to the web browser. This talking needs clear rules called the WebDriver Protocol. When your Selenium script asks the browser to do something, like click a button or go to a URL, it uses this protocol to give the command. If the browser doesn’t respond or runs into an error while doing this, it shows a WebDriverException.
To understand what happened, read the error message that shows up with it. This message can give you useful hints about the problem. To help you understand, we’ve listed the most common causes of WebDriver Exception
Common Causes of WebDriverException
WebDriverExceptions often happen because of simple mistakes when running tests. Here are some common reasons:
- Invalid Selectors: If you use the wrong XPath, CSS selectors, or IDs, Selenium may fail to find the right element. This can create errors.
- Timing Issues: The loading time of web apps often vary. If you try to use an element too soon or do not wait long enough, you could run into problems.
- Browser and Driver Incompatibilities: Using an old browser or a WebDriver that does not match can cause issues and lead to errors.
- JavaScript Errors: If there are issues in the JavaScript of the web app, you may encounter WebDriverExceptions when trying to interact with it.
Why Exception Handling is Important
Exception handling is a crucial aspect of software development as it ensures applications run smoothly even when unexpected errors occur. Here’s why it matters:
- Prevents Application Crashes – Proper exception handling ensures that errors don’t cause the entire program to fail.
- Improves User Experience – Instead of abrupt failures, users receive meaningful error messages or fallback solutions.
- Enhances Debugging & Maintenance – Structured error handling makes it easier to track, log, and fix issues efficiently.
- Ensures Data Integrity – Prevents data corruption by handling errors gracefully, especially in transactions and databases.
- Boosts Security – Helps prevent system vulnerabilities by catching and handling exceptions before they expose sensitive data.
Validating WebDriver Configurations
Before you click the “run” button for your test scripts, double-check your WebDriver settings. A small mistake in these settings can cause WebDriverExceptions that you didn’t expect. Here are some important points to consider:
- Browser and Driver Compatibility: Check that your browser version works with the WebDriver you installed. For the latest updates, look at the Selenium documentation.
- Correct WebDriver Path: Make sure the PATH variable on your system points to the folder that has your WebDriver executable. This helps Selenium find the proper browser driver to use.
Practical Solutions to WebDriverException
Now that we’ve covered the causes and their Important, let’s dive into practical solutions to resolve these issues efficiently and save time.
1.Element Not Found (NoSuchElementException)
Issue: The element is not available in the DOM when Selenium tries to locate it.
Solution: Use explicit waits instead of Thread.sleep().
Example Fix:
WebDriverWait wait = new WebDriverWait(driver, Duration.ofSeconds(10)); WebElement element = wait.until(ExpectedConditions.visibilityOfElementLocated(By.id("elementID")));
2.Stale Element Reference (StaleElementReferenceException)
Issue: The element reference is lost due to DOM updates.
Solution: Re-locate the element before interacting with it.
Example Fix:
WebElement element = driver.findElement(By.id("dynamicElement")); try { element.click(); } catch (StaleElementReferenceException e) { element = driver.findElement(By.id("dynamicElement")); // Re-locate element element.click(); }
3.Element Not Clickable (ElementClickInterceptedException)
Issue: Another element overlays the target element, preventing interaction.
Solution: Use JavaScript Executor to force-click the element.
Example Fix:
WebElement element = driver.findElement(By.id("clickableElement")); JavascriptExecutor js = (JavascriptExecutor) driver; js.executeScript("arguments[0].click();", element);
Related Blogs
4.Timeout Issues (TimeoutException)
Issue: The element does not load within the expected time.
Solution: Use explicit waits to allow dynamic elements to load.
Example Fix:
WebDriverWait wait = new WebDriverWait(driver, Duration.ofSeconds(15)); WebElement element = wait.until(ExpectedConditions.elementToBeClickable(By.id("button")));
5.No Such Window (NoSuchWindowException)
Issue: Trying to switch to a window that does not exist.
Solution: Use getWindowHandles() and switch to the correct window.
Example Fix:
String mainWindow = driver.getWindowHandle(); Set<String> allWindows = driver.getWindowHandles(); for (String window : allWindows) { if (!window.equals(mainWindow)) { driver.switchTo().window(window); } }
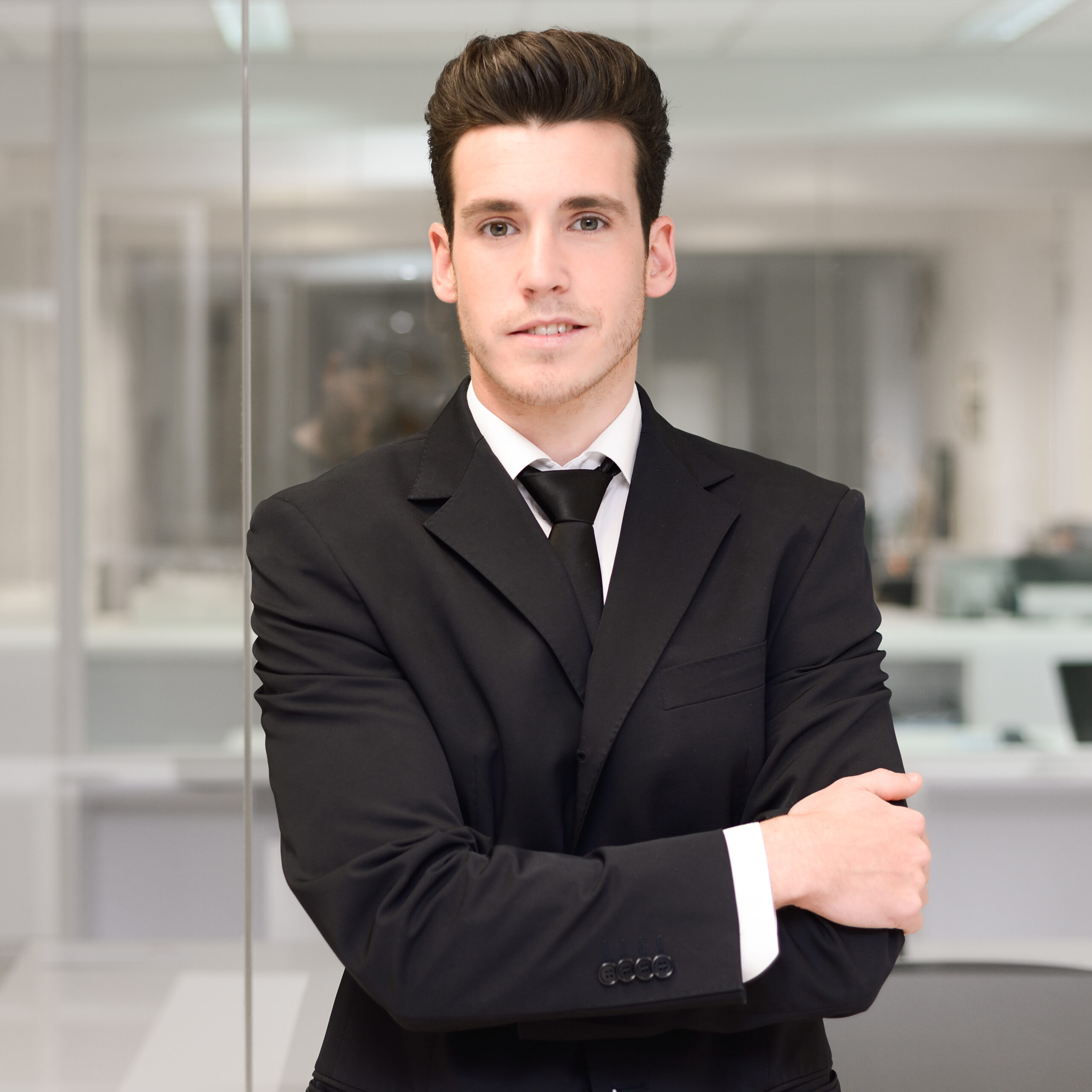
Struggling with WebDriverException in Selenium? Our experts can help you fix it quickly and optimize your scripts!
6.Browser Crash (WebDriverException)
Issue: The browser crashes or unexpectedly closes.
Solution: Use try-catch blocks and restart the WebDriver session.
Example Fix:
try { driver.get("https://example.com"); } catch (WebDriverException e) { driver.quit(); WebDriver driver = new ChromeDriver(); // Restart browser session driver.get("https://example.com"); }
7.No Such Frame Exception (NoSuchFrameException)
Issue: Attempting to switch to a frame that doesn’t exist or is not yet loaded.
Solution: Ensure the frame is available before switching.
Example Fix:
WebDriverWait wait = new WebDriverWait(driver, Duration.ofSeconds(10)); wait.until(ExpectedConditions.frameToBeAvailableAndSwitchToIt(By.id("frameID")));
8.Invalid Argument Exception (InvalidArgumentException)
Issue: Passing incorrect arguments, such as an invalid URL or file path.
Solution: Validate inputs before using them in WebDriver methods.
Example Fix:
String url = "https://example.com"; if (url.startsWith("http")) { driver.get(url); } else { System.out.println("Invalid URL provided."); }
9.WebDriver Session Terminated (InvalidSessionIdException)
Issue: The WebDriver session becomes invalid, possibly due to a browser crash or timeout.
Solution: Reinitialize the WebDriver session when the session expires.
Example Fix:
try { driver.get("https://example.com"); } catch (InvalidSessionIdException e) { driver.quit(); driver = new ChromeDriver(); // Restart the browser driver.get("https://example.com"); }
10. Window Not Found (NoSuchWindowException)
Issue: Trying to switch to a window that has already been closed.
Solution: Verify the window handle before switching.
Example Fix:
Set<String> windowHandles = driver.getWindowHandles(); if (windowHandles.size() > 1) { driver.switchTo().window((String) windowHandles.toArray()[1]); // Switch to second window } else { System.out.println("No additional windows found."); }
11. WebDriver Command Execution Timeout (UnreachableBrowserException)
Issue: The WebDriver is unable to communicate with the browser due to connectivity issues.
Solution: Restart the WebDriver session and handle network failures.
Example Fix:
try { driver.get("https://example.com"); } catch (UnreachableBrowserException e) { driver.quit(); driver = new ChromeDriver(); // Restart WebDriver session driver.get("https://example.com"); }
12.Element Not Interactable (ElementNotInteractableException)
Issue: The element exists in the DOM but is not visible or enabled for interaction.
Solution: Use JavaScript to interact with the element or wait until it becomes clickable.
Example Fix:
WebDriverWait wait = new WebDriverWait(driver, Duration.ofSeconds(10)); WebElement element = wait.until(ExpectedConditions.elementToBeClickable(By.id("button"))); element.click();
or
JavascriptExecutor js = (JavascriptExecutor) driver; js.executeScript("arguments[0].click();", element);
13.Download File Not Found (FileNotFoundException)
Issue: The test tries to access a file that has not finished downloading.
Solution: Wait for the file to be fully downloaded before accessing it.
Example Fix:
File file = new File("/path/to/downloads/file.pdf"); WebDriverWait wait = new WebDriverWait(driver, Duration.ofSeconds(20)); wait.until(d -> file.exists());
14. Keyboard and Mouse Action Issues (MoveTargetOutOfBoundsException)
Issue: The element is outside the viewport, causing an error when using Actions class.
Solution: Scroll into view before performing actions.
Example Fix:
WebElement element = driver.findElement(By.id("targetElement")); ((JavascriptExecutor) driver).executeScript("arguments[0].scrollIntoView(true);", element); element.click();
15.Permission Denied (WebDriverException: unknown error: permission denied)
Issue: The browser is blocking automation due to security settings.
Solution: Launch the browser with desired capabilities to disable security restrictions.
Example Fix:
ChromeOptions options = new ChromeOptions(); options.addArguments("--disable-blink-features=AutomationControlled"); options.addArguments("--disable-notifications"); WebDriver driver = new ChromeDriver(options);
16.Unexpected Alert Exception (UnhandledAlertException)
Issue: An unexpected pop-up blocks execution.
Solution: Handle alerts using the Alert interface.
Example Fix:
try { Alert alert = driver.switchTo().alert(); alert.accept(); // Accept or alert.dismiss() to cancel } catch (NoAlertPresentException e) { System.out.println("No alert present."); }
17. File Upload Issues (InvalidElementStateException)
Issue: Attempting to upload a file but the input[type=”file”] element is not interactable.
Solution: Directly send the file path to the input element.
Example Fix:
WebElement uploadElement = driver.findElement(By.id("fileUpload")); uploadElement.sendKeys("/path/to/file.txt");
18. JavaScript Execution Failure (JavascriptException)
Issue: JavaScript execution fails due to incorrect syntax or cross-origin restrictions.
Solution: Validate the JavaScript code before execution.
Example Fix:
try { JavascriptExecutor js = (JavascriptExecutor) driver; js.executeScript("console.log('Test execution');"); } catch (JavascriptException e) { System.out.println("JavaScript execution failed: " + e.getMessage()); }
20. Browser Certificate Issues (InsecureCertificateException)
Issue: The test is blocked due to an untrusted SSL certificate.
Solution: Disable SSL verification in browser settings.
Example Fix:
ChromeOptions options = new ChromeOptions(); options.setAcceptInsecureCerts(true); WebDriver driver = new ChromeDriver(options);
Advanced Techniques to Resolve Persistent Issues
- If you are dealing with hard to fix WebDriverExceptions, you can try these advanced methods.
- Debugging with Browser Developer Tools: Press F12 to open your browser’s tools. This tool helps you see the web page’s HTML. You can also check network requests and read console logs. Look for errors that might stop WebDriver actions.
- Network Traffic Analysis: If you think there are network issues, use tools to watch network traffic. These tools show the HTTP requests and responses between your test script and the web browser. They can help you find problems like delays, server errors, or wrong API calls.
- Leveraging Community Support: Feel free to ask for help from the Selenium community. You can find useful information in online forums, Stack Overflow, and the official Selenium documentation. This can help you fix many WebDriverExceptions.
Conclusion
In summary, it’s very important to know how to understand and deal with Selenium exceptions, especially WebDriverException. This will help make automated testing easier. First, you should know what the exception means. After that, look at the common causes of the problem. You can avoid issues by checking your setup and keeping everything updated. Use simple ways to troubleshoot and some advanced tips to fix problems well. Stay informed and update your tools regularly to make your testing better. With these helpful tips, you can get your WebDriver to run better and faster. For more help and detailed advice, check out our Frequently Asked Questions section.
Frequently Asked Questions
-
What should I do if my browser crashes during test execution?
– Catch WebDriverException using a try-catch block.
– Restart the WebDriver session and rerun the test.
– Ensure the system has enough memory and resources. -
What are advanced techniques for handling persistent WebDriverExceptions?
– Use network traffic analysis tools to inspect HTTP requests.
– Implement retry mechanisms to rerun failed tests.
– Leverage community support (Stack Overflow, Selenium forums) for expert advice. -
What is the most common cause of WebDriverException?
A common reason for WebDriverException is having a bad selector. This could be an XPath, CSS, or ID. When Selenium can’t find the element you want on the page, it shows this exception.
The post WebDriverException Demystified: Expert Solutions appeared first on Codoid.
Source: Read More